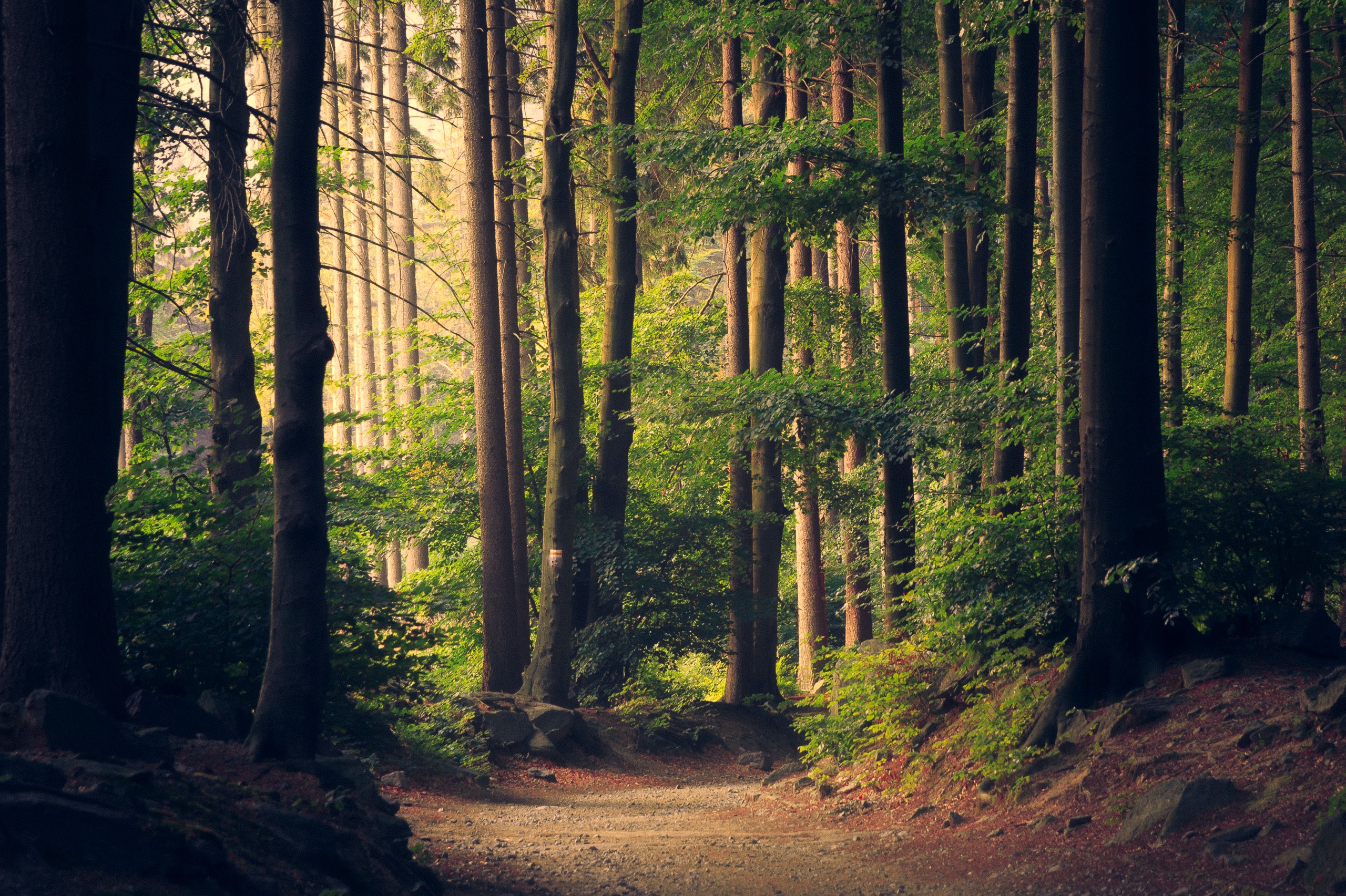
- Test driven development
- Unit testing
Unit Tests as documentation
One of the advantages of doing test driven development & unit testing is having the unit tests as documentation of the code. When you read this in the unit testing / TDD books, it is hard to imagine how this would be of use in practice.
As one of my favorite bloggers, Jeremy Miller, already writes, the NHibernate source is a really good example of using the tests as documentation. I think this is especially the case when developing frameworks or libraries. Because the code will always be used by developers, the best way for a developer to see how the code should be used is by looking at the unit tests as an example.
I experienced this myself a couple of days ago with the same code that Jeremy mentions. I also used the NHibernate EnumStringType to use an enum type that is represented in the database as a string column. This works great, but when I wanted to use the enum type in a HQL query, it didn’t work the way I had expected:
IQuery q = s.CreateQuery("from Order as order where order.State =:orderState"); q.SetEnum("orderState", OrderState.Open); IList results = q.List();
The code above gave me a vague oracle exception. So i needed to figure out how to pass the parameter to the HQL query. I fired up the NHIbernate source to open the unit test for the EnumStringType:
I then opened the test, which gave me the right code:
[Test] public void ReadFromQuery() { ISession s = OpenSession(); IQuery q = s.CreateQuery("from EnumStringClass as esc where esc.EnumValue=:enumValue"); q.SetParameter("enumValue", SampleEnum.On, new SampleEnumType()); q.SetEnum("enumValue", SampleEnum.On); IList results = q.List(); Assert.AreEqual(1, results.Count, "only 1 was 'On'"); q.SetParameter("enumValue", SampleEnum.Off, new SampleEnumType()); results = q.List(); Assert.AreEqual(0, results.Count, "should not be any in the 'Off' status"); s.Close(); // it will also be possible to query based on a string value // since that is what is in the db s = OpenSession(); q = s.CreateQuery("from EnumStringClass as esc where esc.EnumValue=:stringValue"); q.SetString("stringValue", "On"); results = q.List(); Assert.AreEqual(1, results.Count, "only 1 was 'On' string"); q.SetString("stringValue", "Off"); results = q.List(); Assert.AreEqual(0, results.Count, "should not be any in the 'Off' string"); s.Close(); }
As Jeremy also mentions, the NHibernate documentation isn’t as complete as we would have hoped, the EnumStringType isn’t documented for example. But as the example above illustrates, these unit tests can be really helpful as technical documentation, especially when it concerns frameworks and libraries.